Exploring API Endpoints in Depth
At a high level, API endpoints are like middlemen that let different pieces of software talk to one another. They deal with HTTP requests and move data around — and are therefore essential for today's connected Simple enough. But for those making web APIs, it's necessary to have a deep understanding of how they work and what they do.
In this post, we're going to start at square one with API endpoints, covering everything from the kinds of API endpoints and best practices for building them, keeping them secure, and ensuring they run fast.
What are API endpoints?
API endpoints are like the doors to a web service. Through these endpoints, we can enter and talk to a web service and be shown where and how we can gain access to whatever it is the server has. This process allows separate pieces of software to swap information in a controlled way. Understanding the inner workings of API endpoints allows organizations to ensure smooth connections and data exchanges from the server.
API endpoints' role in communication
API endpoints set up routes for different programs to send requests and get back answers. They ensure that data moves smoothly between the user's side (the client) and the place where all the data is kept (the server). By opening access to certain features in an app, API endpoints allow us to ensure that everything works together well within a system.
The anatomy of an API request and response
When someone uses an API, they send a request to it by choosing what kind of action (for example, getting or sending data) they want and where to send it. This is done through something called an endpoint URL. After the API server gets this request, it figures out what needs to be done and sends back a reply over HTTP. This reply tells you if your request worked or not with a status code and gives you the details in JSON format (which is just a way computers share information).
Types of API endpoints
When you're dealing with APIs, it's important to get the hang of the different kinds of API endpoints out there.
First up, we've got RESTful and SOAP endpoints which don't do things the same way. RESTful is all about flexibility and is pretty popular because of that. Then there are GraphQL endpoints, which let you tailor your data queries more precisely. Each kind has its own pros and cons, depending on your needs and priorities. By picking the endpoint that fits your project best, you can ensure everything runs smoothly and makes users happy.
Understanding these differences well helps in making choices that boost user experience while working efficiently with API query options like GraphQL.
RESTful vs. SOAP endpoints: A comparative analysis
When we look at RESTful versus SOAP endpoints, some clear distinctions stand out. For starters, RESTful APIs are more streamlined and use URL structures to carry out actions, making them a popular choice for web apps. On the flip side, SOAP endpoints stick to a stricter format by using XML messaging and WSDL for their communication needs. With its stateless nature, RESTful can work quicker but when it comes down to security levels, SOAP takes the lead with its WS-Security standards. Knowing these subtle differences is key in picking the right one depending on what your project needs.
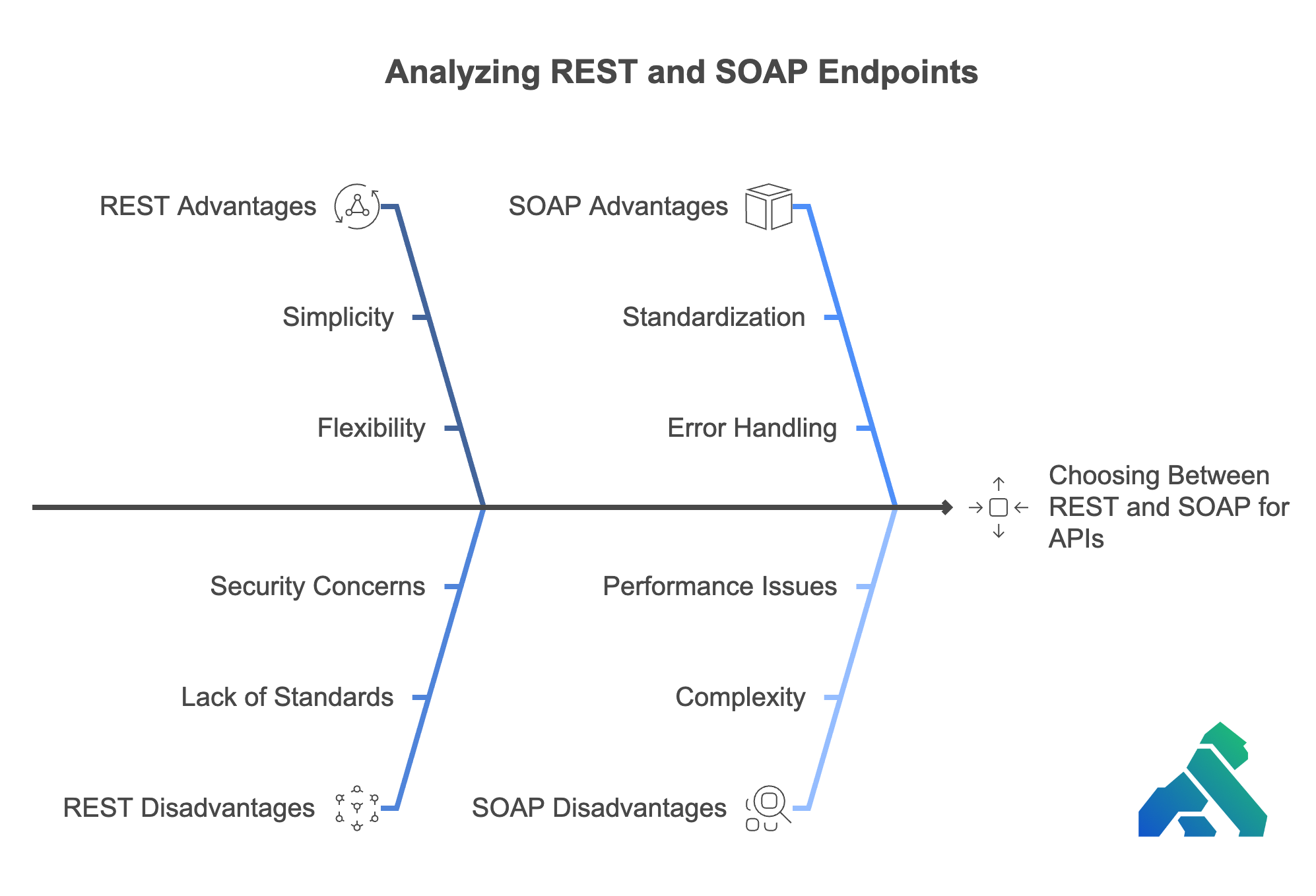
Understanding GraphQL endpoints
With GraphQL endpoints, clients have the freedom to ask for just the data they want. This cuts down on grabbing too much info, a common problem with REST APIs. Instead of needing different spots (endpoints) for various types of information like in REST, GraphQL lets you pull together requests for all sorts of data from one place. By doing this, it works faster and makes getting your hands on the right data easier. Plus, thanks to its schema that looks inward (introspective), GraphQL offers strong and accurate ways to query what you need.
How to design effective API endpoints
When you're setting up API endpoints, it's important to stick to naming rules that are (a) easy to understand and (b) remain the same across the board. This makes everything clearer for people trying to read through them.
Organize endpoints in a way where they're grouped by what they do. This helps everyone find their way around more easily. Make sure security is at the top of your list by using strong ways for users to prove who they are and checking data carefully. Write down lots of details about your APIs so developers know how to work with them right off the bat. Think about making sure your design can handle growing without too many hitches, focusing on keeping things running smoothly and quickly from the start.
By regularly checking over your endpoint setup against what works best and what users need, you'll make sure it stays on track.
Adding all these pieces together really boosts how well an API works overall and improves user experience significantly.
Best practices for endpoint structure and naming conventions
When it comes to naming, it's key to stick with naming rules that are easy for everyone to understand and follow going forward. Ideally, names should be short but clear — explaining what they do and making it obvious which resources they're related to. By adding versioning in the endpoint URL, you can handle updates without a hitch. It's smart to make your endpoints straightforward and directly linked to their tasks. It sounds simple enough, but it's true: basically, avoid making things too complicated. This makes things easier for developers and takes some of the pains out of growing.
Everything should be clear-cut and work well together in your API design. Ensuring a consistent way of operating also makes writing documentation simpler — and working together smoother.
Security considerations in API endpoint design
Security is an essential component to keep in mind when you're setting up API endpoints. There are a few considerations to help ensure security.
- Consider using strong methods to check who's accessing your API, like OAuth or API keys.
- Make sure data sent over the internet is safe by using HTTPS and SSL certificates.
- Keeping tabs on what people input helps stop bad code from getting in.
- Putting limits on how much people can use your service can prevent anyone from overloading it and keep things running smoothly.
As with so many things in this digital world, it's a good idea to regularly check for security problems and keep an eye on things to catch any issues early on.
By focusing on these points when designing your endpoint for an API, you protect sensitive information and make the whole system more reliable with features like authentication, rate limiting, and input validation.
How to implement API endpoints
Using REST or GraphQL servers are common and established approaches for building APIs. You can start by setting up routes and figuring out how to manage incoming requests and outgoing responses.
For making an API server, especially if you're working with Node.js, frameworks such as Express are handy. Since keeping endpoints secure should always be a top priority, consider standard methods for securing APIs like OAuth and API keys. OAuth is especially clutch for user authentication flows, while API keys are commonly used for communication between services. Keeping documentation clear is another key best practice as proper API documentation is needed for adoption and maintenance. Finally, don't overlook API testing with tools designed for this purpose, like Kong Insomnia or Postman.
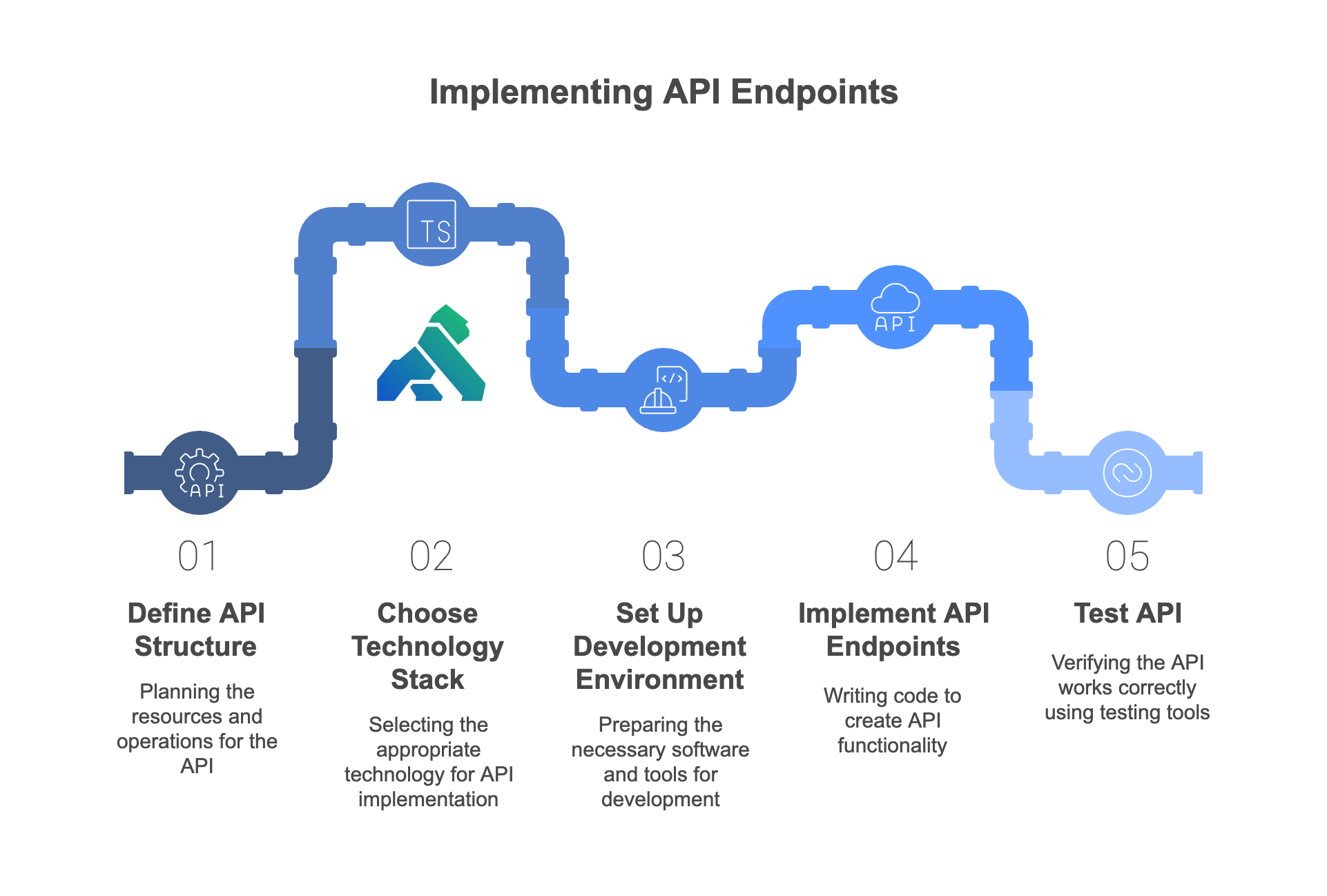
Tools and technologies for building API endpoints
When it comes to creating strong API endpoints, there's a wide array of tools and tech for developers to pick between. Frameworks such as Express.js for Node.js or Django for Python can ensure the process of making an endpoint is smoother. API testing tools can come in handy to check if the endpoints are working properly. When it comes to saving data, options like MySQL or MongoDB can come in handy. For keeping everything clear about the API, there are also tools to help with documentation. To keep things safe, JSON Web Tokens (JWT) and OAuth are used to protect the endpoints. On top of that, monitoring tools are there to make sure these points run well by tracking their performance. All these various tools play a big part in putting together APIs that do what they're supposed to and stay secure.
Step-by-step guide to developing your first API endpoint
First, figure out what your API is going to do. Think about the types of data it'll handle and sketch out the main URL for your API. Next, decide which HTTP methods you're going to use and plan how your endpoint URLs will look. With that in place, make sure you add some security steps like using API keys for authentication. It's also important to write down clear instructions to document how others can use your API properly. Before you let anyone else try it, give all parts of your endpoint a good test run yourself.
Securing API endpoints
Strong login checks and permissions are foundational to keeping your API endpoints secure. Things like rate liming, SSL encryption, and token-based logins can tighten up your endpoint security. Keeping tight control around who can access can help prevent common dangers like DDoS attacks and data leaks. Don't forget to change API keys and passwords frequently, and use API monitoring to keep an eye out for any unusual behavior.
Authentication and authorization mechanisms
Authentication and authorization play a huge role in keeping API endpoints safe. Authentication lets you see who's knocking at the door. It's like asking for ID before letting someone into your club. Authorization, on the other hand, is used to determine what level of access you have. Can you enter the VIP area? How about the manager's office?
With OAuth, clients can grab access tokens that act as their entry badges, proving who they are without giving away passwords or other secrets. API keys are like special passes given out that need to be shown every time you make an API request.
Having strong authentication and authorization processes in place that follow established best practices and rules (like those set by OAuth) help to ensure that only the right people can talk to your API. This keeps things running smoothly — without any unwanted guests causing trouble.
Mitigating common security threats to API endpoints
Making sure API endpoints are safe helps to keep important data secure and stops people who shouldn't have access from getting in. There are some common security risks to your API endpoints, and it's important to put plans in place to deal with them
- Distributed Denial of Service (DDoS) attacks occur when the API server is flooded with so many requests that it can't handle them all and stops working properly. Fight these kinds of attacks off by implementing things like rate limiting (which controls how much traffic comes in) or shaping the traffic better. You may even consider using services designed to stop DDoS attacks.
- Making sure the data sent between the API client and server is super secure matters too. Using SSL/TLS encryption helps to ensure prying eyes can't sneak a peek at your data on its journey from point A to B.
- Strong passwords rules around your API endpoints and adding extra layers like multi-factor authentication are important for fending off many common threats. Authentication can make it so that even if someone gets hold of a password somehow; they still need another way to prove who they say they are before getting access.
- Keeping everything up-to-date — including both the main software running on your servers as well as any other bits it relies on — helps keep known bugs away that could let attackers through.
By putting these steps into action developers will make their APIs much safer against different types of threats out there.
Testing and monitoring API endpoints
By testing and monitoring API endpoints properly, developers can spot and sort out problems before they bother users — and ensure they work well, are fast, and are safe.
With API testing, it's all about creating detailed tests that look at different situations. Test each endpoint on its own, pay attention to the status code coming back, and make sure the data going in is correct.
Monitoring these endpoints lets us see how healthy they are, what their speed is, and if they’re up or down. There are many tools out there for this job that give real-time updates on things like how long responses take, user experience, and uptime.
Thorough testing and vigilant monitoring ensure your API consumers get a great experience, while also looking after the wellbeing of your entire API setup.
Strategies for effective API testing
Effective API testing requires a comprehensive approach that combines end-to-end testing, careful data management, and various test types including contract, performance, and security testing.
A robust testing strategy should incorporate automation best practices, such as parameterized tests and continuous integration, while maintaining separate test environments and consistent data seeding practices. For reliable results, it's crucial to implement both happy path and edge case scenarios, monitor test coverage and execution times, and ensure proper test documentation and maintenance over time.
Tools for monitoring API endpoint health and performance
Keeping an eye on how well API endpoints perform helps us to ensure they're working right and giving users a smooth experience. For these purposes, there are a variety of tools available that help us keep track of endpoints, showing how they're performing and if they're available when needed. Monitoring tools let developers get ahead of problems by spotting them early.
Troubleshooting common API endpoint issues
Ensuring API endpoints function correctly helps to allow for optimal performance and user experience. Common issues may include connectivity problems, often due to network issues, firewall configurations, or server-side errors. Addressing these requires checking network connections, firewall settings, and server configurations. Data format errors, on the other hand, require robust input validation to ensure only correct and meaningful data is processed.
Efficiently resolving these issues enhances API reliability and overall performance.
Diagnosing connectivity problems
Identifying and resolving connectivity issues is essential for smooth API operation. Problems may stem from network disruptions, incorrect server setups, or restrictive firewall rules. Developers typically employ diagnostic tools like ping or traceroute to trace network problems and scrutinize API server configurations to uncover any mismatches. Proactively addressing these issues is key to maintaining seamless API communication and performance.
Handling data format and validation errors
Correct data format and validation are pivotal for proper API functionality. Errors often occur when the client sends data in an incorrect format or with invalid content. Implementing stringent validation checks ensures data integrity and correct formatting, preventing errors downstream. Additionally, adapting data to meet endpoint requirements, such as converting JSON to XML, is crucial for smooth data handling.
Optimizing API endpoint performance
Enhancing API endpoint performance is vital for a positive user experience, focusing on response times and scalability. There are several ways to make this happen and to ensure quick response times.
Strategies include optimizing code, improving database queries, and implementing caching for frequently accessed data. To manage increased traffic, load balancing across servers and rate limiting are effective. These measures ensure fast, reliable, and scalable API endpoints, crucial for user satisfaction.
Then there's rate limiting which helps keep things under control by setting limits on how many requests someone can make in a certain period of time. This is like putting up speed bumps to prevent traffic jams and ensure everyone has fair access without crashing the system.
By focusing on speeding up responses, managing heavier loads efficiently, and controlling traffic flow with rate limiting, developers can create API endpoints that are fast, reliable, and able to handle growth gracefully — all contributing positively towards an awesome user experience.
Techniques for reducing latency
Minimizing latency is key to improving user experience with API endpoints — al ong wait after asking for something from an API endpoint is not a positive user experience. Thaknfully, there are a few ways we can make this process run at optimal speeds.
Efficient API design, including pagination, filtering, and sorting, reduces unnecessary data exchanges. Caching frequently requested data and employing efficient network protocols further decrease response times. These strategies collectively aim to reduce latency, ensuring faster and more reliable API responses.
Scaling your API for high traffic
Preparing your API to handle high traffic involves ensuring scalability and uninterrupted service. Horizontal scaling distributes the load across multiple servers, while rate limiting prevents any single user from overloading the system. Strengthening backend components, like databases and caches, ensures they withstand traffic surges. These approaches help maintain performance and service quality, even during peak usage periods.
API Endpoints FAQs
What are API endpoints and why are they important?
API endpoints act as communication gateways that allow different software applications to interact with each other. They're crucial because they manage HTTP requests and data transfer, enabling seamless communication between clients and servers. Well-designed endpoints are essential for modern websites and applications to function effectively.
What are the different types of API endpoints?
There are several main types of API endpoints, including RESTful, SOAP, and GraphQL endpoints. RESTful endpoints are known for their flexibility and popularity, SOAP endpoints offer robust security through WS-Security standards, and GraphQL endpoints allow precise data querying from a single endpoint. Each type has unique advantages depending on your project requirements.
How can I secure my API endpoints?
Secure your API endpoints by implementing strong authentication and authorization mechanisms like OAuth or API keys, using SSL/TLS encryption for data transfer, and enforcing input validation. Additionally, implement rate limiting to prevent DDoS attacks, regularly update security measures, and monitor for suspicious activities.
What are the best practices for API endpoint design?
Follow clear naming conventions and maintain consistency across endpoints. Group endpoints logically by function, implement proper versioning in URLs, and keep the structure simple and intuitive. Document everything thoroughly and design with scalability in mind to ensure your API can grow without issues.
How can I optimize API endpoint performance?
Optimize performance by implementing efficient caching strategies, using pagination for large data sets, and optimizing database queries. Consider horizontal scaling for high traffic, implement rate limiting to manage resource usage, and use load balancing to distribute traffic effectively across servers.
How do I troubleshoot common API endpoint issues?
Address common issues by first checking connectivity problems through network diagnostics, verifying server configurations, and reviewing firewall settings. For data format errors, implement proper validation checks and data transformation processes. Regular monitoring and logging help identify and resolve issues quickly.
What's the role of authentication and authorization in API endpoints?
Authentication verifies the identity of users or applications accessing the API, while authorization determines their access privileges. These security measures are typically implemented through tokens, API keys, or OAuth protocols to ensure only authorized users can access specific endpoint functionalities.
How can I scale my API endpoints for high traffic?
Scale your API by implementing horizontal scaling across multiple servers, using load balancing techniques like round-robin or weighted distribution, and setting up proper rate limiting. Strengthen your infrastructure by optimizing database performance and implementing efficient caching strategies to handle increased traffic loads.
What should I consider when implementing API endpoint monitoring?
Focus on monitoring key metrics like response times, error rates, and endpoint availability. Use monitoring tools that provide real-time alerts, performance dashboards, and detailed analytics. Regular monitoring helps identify potential issues before they impact users and ensures consistent API performance.
Conclusion
Understanding API endpoints means understanding how software "talks" Setting up endpoints properly and making sure they're locked down tight with good security checks are key. The little decisions made throughout the process matters when it comes to making APIs that work well and fast. By sticking to the best practices, keeping an eye on how things are running, and promptly addressing any usual problems, your API endpoints will be more reliable and can handle more stuff without breaking a sweat.
The API-First Journey Starts Here: Become a secure, API-centric enterprise
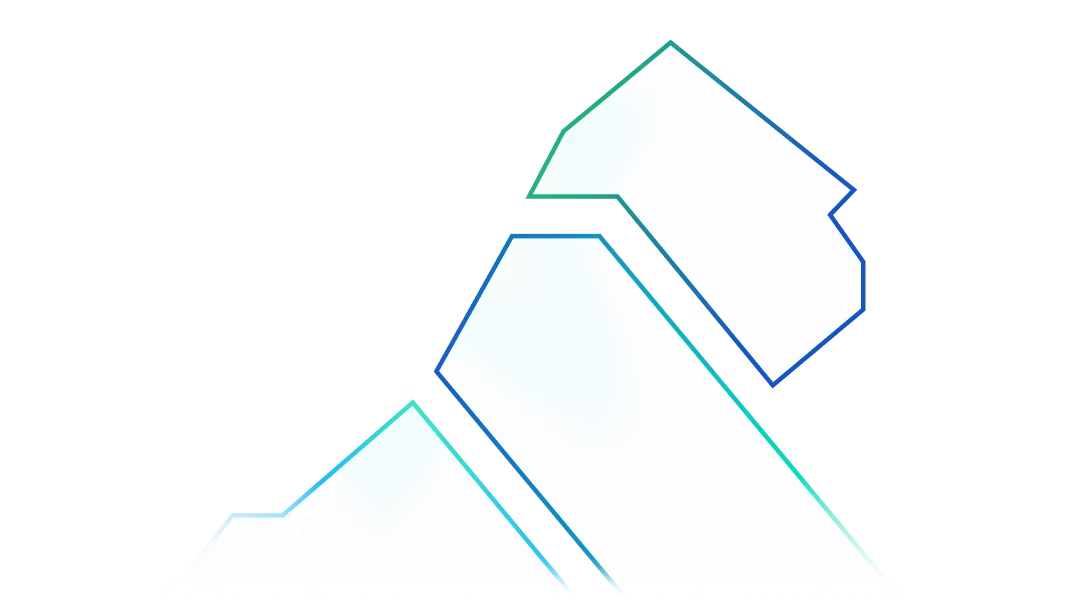