What is a REST API? A Comprehensive Guide
A RESTful API is a type of application programming interface that follows the guidelines of Representational State Transfer (REST). Its goal is to present data models and functions in a clear and standard format. RESTful APIs use common web technologies. This helps them work smoothly across different platforms. Many people prefer these APIs for web and mobile apps. They are simple to use and can expand with your needs. This makes them a great option. In this article, we will learn more about RESTful APIs, how to use them, and other important things to know.
What are REST APIs?
REST means Representational State Transfer. It is a method to design web APIs. REST uses standard HTTP methods to fetch information from resources using URLs. Here are some key rules for REST API design:
- You can find resources using URLs. This helps clients access and change them with HTTP methods like GET, POST, PUT, and DELETE. Resources can be documents, images, or other items that the service manages.
- Representations of resources are usually shared in JSON or XML format. These representations show the resource's status at the time of the request.
- The communication is stateless. This means the server does not keep any client information between requests. Each request has all the information to handle it. This makes the system more efficient and reliable.
- Standard HTTP methods, such as GET, POST, PUT, and DELETE, manage resources in a clear way. This makes it easier to implement.
- Clients receive clear error messages. This helps them fix issues without needing more information from other sources.
How do RESTful APIs work?
RESTful principles
RESTful APIs follow certain rules. One rule is that they have a uniform interface. They are stateless, which means they do not have to remember old requests. Their URIs often look like a directory. They can also send resources in different formats, like XML or JSON.
In an API, everything you can use or reach is displayed as an HTTP resource. This resource is found at a URI endpoint. URIs help to name groups, single items, attributes, and more.
HTTP methods
REST uses familiar HTTP methods to work with resources. GET is for getting data. POST is used to create new resources. PUT updates resources that already exist. DELETE takes away resources.
Clients send requests to REST API endpoints. These requests come with HTTP headers. The headers provide key details such as authorization tokens, the type of content, and acceptable formats. Parameters help filter results or connect different pieces of data.
A typical REST endpoint would look like:
GET https://api.example.com/users/1234
The user with ID 1234 is the key resource here. The base URL reveals the location of the api server. The users path indicates that this is a user resource.
Common patterns for REST endpoint URLs:
- /users - Handle accounts for users
- /posts - See blog posts
- /devices/{deviceId} - Control single IoT devices
- /reports?type=sales - Sort the report collection
Endpoints use URL paths and queries. This shows how to get to data easily.
Servers send HTTP response codes to show the results. They also add response headers to explain the content. The message body contains details about resources or data.
Statelessness means that each RESTful request is independent. Each request does not rely on any server information or previous actions. Sessions are managed with tokens.
Caching support
REST APIs speed things up by saving copies of resources on the server or client. This can happen because HTTP lets us use caching.
Self-documentation
REST APIs use HTTP rules for response codes, actions, and media types. This means that the APIs show clients how to use them.
REST API analogy for the non-technical
Think of a REST API like a menu at your favorite restaurant. Each dish represents a resource, like an image or a document. When you choose from the menu, you use HTTP methods to place your order. The kitchen, which acts as the server, prepares your dish based on what you asked for.
Clients can easily order, update, or delete items from the menu. They can also manage resources using HTTP methods such as GET, POST, PUT, and DELETE. The great thing about this system is that it is organized. You can access it with simple actions. This makes it easy for both people and machines to use!
REST vs SOAP
SOAP and REST build web services in different ways. SOAP is a protocol with strict rules for XML messaging. It describes how services, transactions, and security should work. You use structured XML to make requests and get responses for operations. SOAP services are explained clearly with WSDL documents. These documents give detailed data about each action. This helps make robust tools, but it can also feel complicated.
REST is a way to design systems. It follows certain principles, like having a uniform interface and interactions that do not depend on each other. With REST, people can work with resources using URIs. They can also move resource data, like JSON and XML, between clients and servers. Instead of showing different operations, REST lets you access named resources, much like you would open files in a folder. By focusing on architectural constraints for better scalability, REST wants to make connections simpler and lighter.
REST is now a popular choice for mobile and web apps that connect to microservices. This is mainly because it is easy to use and works well with JSON. REST allows for quicker development and consumes less bandwidth. Although SOAP has complex tools that can be better for difficult business tasks, many people prefer REST these days. For effective and widespread use, REST meets the needs of modern apps. It aims to be simple and can expand its reach to a large online audience.
How is a RESTful API different from other APIs?
Architectural style
REST is a way to design software. It follows specific rules. For example, it has a uniform interface and is stateless. REST uses resources and representations. Other API methods, such as RPC or SOAP, are less organized and do not follow a specific style.
REST serves as an interface that uses important parts of the web. This includes HTTP methods, URIs, headers, body, and status codes. This design helps clients access the resources they want. In contrast, RPC APIs provide several custom methods. These methods are a better fit for how apps function internally.
In REST, we see resources as nouns. Each item has a unique URI and popular endpoint paths. Resources can be one noun or a collection of nouns. In contrast, RPC is all about using verbs or functions to change data directly.
Statelessness means that REST considers each request separately. It does not remember the session state between messages. This approach makes it more scalable and efficient. In contrast, RPC protocols do remember the state from different requests but are harder to scale horizontally.
- Caching Support: We can improve REST performance by using built-in HTTP caching. This is effective because each request stands alone.
- Custom RPC protocols require specific caching to achieve the best outcomes.
Self-descriptive messages
REST uses status codes, MIME types, and headers. These tools help responses show metadata. This metadata tells you about the format of the payload, any errors, and more. In contrast, RPC protocols put this metadata in the message arguments. This means you need special parsing to read the information.
REST creates guidelines for using key web technologies in architecture. This makes it simpler and more effective for clients and servers to interact, especially when dealing with large systems. In contrast, RPC does not emphasize having a standard approach. Instead, it highlights how applications operate internally.
What are the benefits of RESTful APIs?
Simplicity
REST APIs use HTTP. They follow standard methods and error codes. This helps clients connect easily to web services. Clients do not need complex libraries or tools. A stateless request model makes it easy to scale the server. This combination creates a simple experience for clients, servers, and networks during integration.
Flexibility
REST gives you the choice of data formats. You don’t have to use just XML or JSON. You can also send resource information in plain text. This flexibility lets developers be creative with how they design resources. They can pick different ways to package the data. This approach allows development to change and grow over time.
Scalability
The stateless request model means each request has all the information needed. This makes REST interactions easy to scale. You can add more servers without the worry of sharing state between them. This way, it can handle many internet users and mobile devices well.
Performance
- Built-in caching support helps to make performance better and reduces the load on servers.
- Content delivery networks can keep data closer to users.
- Many domains can handle REST APIs separately. This makes systems stronger.
Portability
REST uses standard HTTP and JSON. This makes it simple to use with many programming languages and platforms. Examples include JavaScript, Java, .NET, Android, and iOS. It helps create systems where different parts can be built with different languages.
Protect Mission-Critical APIs & Services: Efficient protection strategies revealed
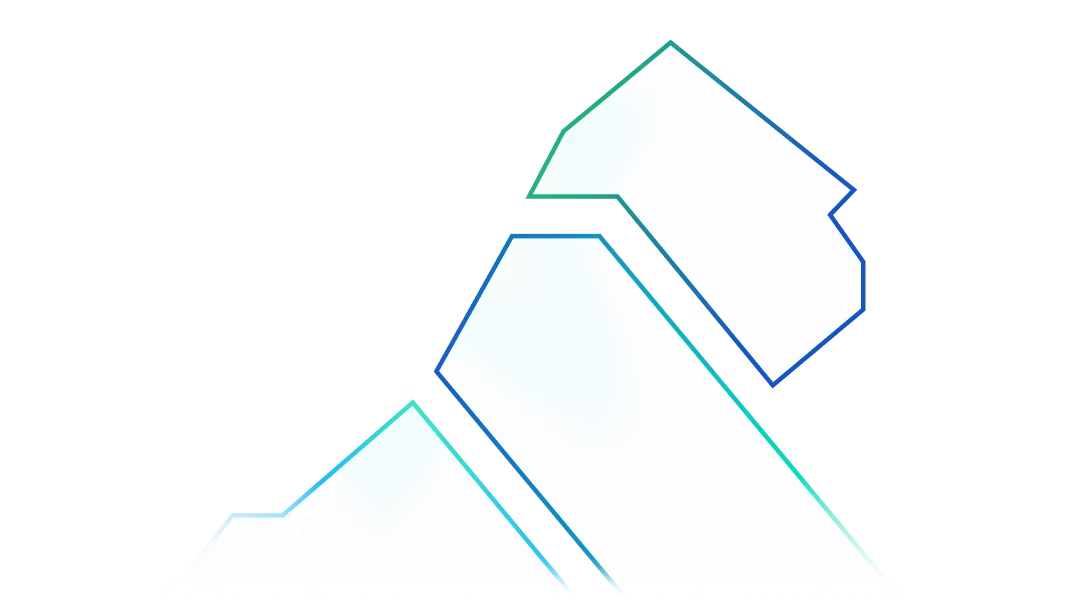
What are some real-world examples of RESTful APIs?
RESTful APIs are used a lot in web and mobile apps. They help you get or change resources and data in other systems. Here are some examples:
- Social media sites, like Twitter and Facebook, use REST APIs to connect with other apps. They allow users to post updates.
- Ridesharing apps, such as Uber and Lyft, use REST APIs. They help manage cars, access maps, fares, and location details.
- Streaming services, like Netflix and Spotify, rely on REST APIs. They access information about media files from servers.
- Banking apps also use REST APIs. They help you get your account data and start transactions with remote servers.
- IoT apps connect with sensors and devices through REST APIs. This lets them check the devices and send commands.
Overall, REST is good for linking new apps to web services. It is simple to use and easy to expand.
REST API security practices
REST APIs help keep things secure and in order. They do this by allowing safe access to resources through authentication methods. For instance, authorization tokens are included in HTTP headers. This way, only users who have permission can use the API endpoints. It helps protect data and keeps it private.
Parameterizing requests helps to narrow down results according to what users enter. This improves safety by stopping unauthorized people from getting to sensitive data. By using common URL patterns and query parameters, developers can make RESTful APIs that are simple and secure. This follows the best practices in the industry.
Statelessness in RESTful architecture reduces issues that come with sessions. It also makes it easier to expand the system.
What are RESTful API authentication methods?
There are many common ways to use authentication and authorization in RESTful APIs.
- API keys - You send an API key in the request header. This helps the server know who is making the request. It is simple to use, but it is not very safe.
- basic auth - You can put your username and password in an Authorization header. The server decodes and checks this information. Since it is sent without protection, the security is low.
- OAuth 2.0 - This is an open standard that uses tokens to show who the user is and what they can do. It allows users to access the system safely.
OpenID Connect is a way to manage identity. It works with OAuth 2.0. You can log in with accounts from different providers, such as Google or Facebook. This helps make logging in simpler and more secure.
Certificate-based systems, such as mutual TLS authentication, make use of digital certificates. These certificates help confirm the identity of both the client and server machines.
The role of middleware in REST API integration
A main part of understanding REST API security is knowing how Middleware plays a role. Middleware is crucial for making REST API interactions safe and dependable. It acts like a bridge between the client application and the server. It helps with several jobs, including authentication, logging, changing requests and responses, and managing errors.
Using middleware in REST API integration helps developers add security features. These features are access control, input validation, and encryption to protect against attacks. Middleware also helps communication flow better between different parts of the system. This improves performance and makes everything more reliable.
RESTful API design and architecture constraints
When developers create REST APIs, they must follow certain standard rules. This practice helps maintain a RESTful design.
- Client-Server: There is a clear gap between the API client and the server. This practice helps to organize the interface better.
- Statelessness: The server does not remember client details between requests. Each request has all the information needed. This makes it easier to grow and update the server. Requests should be simple, if possible.
- Cacheability: REST responses should have rules that show which resources can be cached. Caching helps the system work faster and stay strong. Also, versioning helps to stop changes that might break the client.
- Uniform Interface - By using standard HTTP actions such as GET, POST, PUT, and DELETE, the API interface remains consistent for all services. Resources have unique identifiers called URIs.
A layered system has load balancers in its design.
- It has parts like firewalls and load balancers.
- This setup does not change how clients speak to servers.
- It helps to make things bigger and better.
How to build and implement RESTful APIs
A standard way to create a new RESTful API service includes:
- Find out what the API needs to show. It should be based on how the application will be used. Resources are real things like accounts and product catalogs.
- Give each resource a unique ID. You can do this by making URLs like /users or /accounts. Resources can also have a structure, like /customers/1234/orders.
- Use common HTTP methods to work with resources. For example, use GET to get data, POST to create data, and DELETE to remove resources.
- Choose a data format for sharing information. You can use JSON or XML. JSON is the most popular choice.
- Create a design for how api requests and responses should look, often for JSON data. You can use schemas, like OpenAPI Specification, to explain them clearly.
- Build resource route handlers using a programming language like Node.js or Java. These should handle tasks like getting data from a database and sending back nicely formatted responses.
- Make sure to add authentication to the API for better security.
- Set limits on how often users can access the API.
- Use caching to make responses quicker and lessen the load on the server.
- Offer clear documentation so users can easily learn how to use the API.
- Check the API interface thoroughly before it launches.
- Collect user feedback to make improvements later.
Summary
RESTful web APIs offer a simple way to build web service interfaces that can easily grow. They focus on main resources and use common HTTP methods. This approach helps in creating connected apps for both mobile devices and the web. A good RESTful design is important for today’s APIs because it comes with powerful tools and can handle development. Plus, REST's flexibility allows APIs to stay useful as needs change over time.