The Ultimate CORS Crash Course
In today's interconnected web ecosystem, modern applications frequently need to communicate across different domains, making Cross-Origin Resource Sharing (CORS) a fundamental concept for web developers to master. This comprehensive guide explores CORS from its basic principles to advanced implementation strategies, helping developers understand how to enable secure cross-origin communications in their applications. Whether you're building a microservices architecture, integrating third-party APIs, or developing a distributed web application, understanding CORS is crucial for maintaining security while enabling necessary cross-origin interactions.
What is Cross Origin Resource Sharing (CORS)?
Cross-Origin Resource Sharing (CORS) is a security tool that helps web servers decide who can use their resources. By default, web browsers enforce the "Same-Origin Policy," which prevents a webpage from making requests to a different domain than the one serving the webpage. This is a security measure to prevent malicious websites from making unauthorized requests to other domains on behalf of users. Knowing about CORS is important for web developers. CORS is like a security checkpoint that controls which websites can talk to each other. Think of it this way: When you're on Website A, and it needs information from Website B, CORS is the security guard that decides whether Website A is allowed to get that information or not.
Real World CORS Analogy
Imagine you're visiting a bank. When you walk in, you need to show your ID to prove who you are. This is like the security check that happens on the internet.
Now, let's say you want to get money from your account at Bank A, but you're physically at Bank B. Bank B will need to:
- Check if you're allowed to access Bank A's services
- Call Bank A to verify if they allow transactions from Bank B
- Get permission from Bank A before helping you
CORS works in a similar way on the internet. When you're on one website (let's say Facebook) and that website needs to fetch something from another website (like PayPal for a payment), your web browser acts like a security guard:
- It first checks with PayPal: "Hey, Facebook is trying to access your services. Do you allow this?"
- PayPal then needs to explicitly say "Yes, Facebook is allowed to interact with us" or "No, we don't allow this"
- If PayPal says no, your browser blocks the connection to keep you safe
This whole process is like having a security guard (your web browser) checking if one building (website) has permission to access resources from another building (website). It's a safety measure to protect your information and prevent malicious websites from stealing data from other websites you use.
Without this security system, it would be like allowing anyone to walk into any bank and access any account without any verification – which wouldn't be very safe!
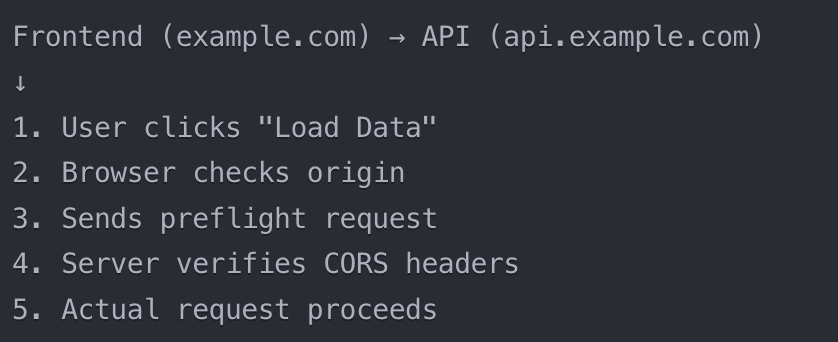
CORS Core Concepts
Before we dive into the technical stuff, let's cover some basic CORS ideas. Knowing these ideas is key to understanding how CORS works and how to use it well.
CORS mainly involves a web browser and a web server. When a browser asks a server for a resource, it sends details about its origin. This includes the domain name, the type of connection (like HTTP or HTTPS), and the port number. The server then looks at its CORS configuration to decide if it should allow or deny the request. This choice is sent back to the browser using specific HTTP headers.
First, What is Same-Origin Policy?
The Same-Origin Policy is a fundamental security feature implemented by web browsers. It restricts how a document or script from one origin can interact with resources from another origin. An origin consists of:
- Scheme (e.g., HTTPS)
- Hostname (domain)
- Port number
For example, https://www.example.com, https://api.example.com, and http://www.example.com are different origins, even though they have the same base domain.
Because of this policy, a web page can only do AJAX requests for resources that come from the same origin as where it was loaded. This means that if a web page comes from https://www.example.com, it can only make requests to resources in the https://www.example.com domain.
This restriction is done through HTTP headers. When a browser makes a request, it adds an Origin header that shows its origin. The server can check this header and decide if it will allow or block the request based on its CORS configuration.
When CORS Comes Into Play
The Same-Origin Policy is important for security. However, it can limit safe interactions between different origins that trust each other. This is where CORS helps.
CORS lets servers specify which origins can access their resources. This makes the Same-Origin Policy a bit more flexible. Servers do this by using special HTTP headers when talking to the browser during a cross-origin request.
When a browser makes a cross-origin request, it first sends a special HTTP OPTIONS request to the server. This is known as a "preflight" request. It asks the server if it can send the real request. The preflight includes details like the HTTP method (GET, POST, etc.) and any custom headers. The server replies with its own CORS headers. These headers tell the browser whether the actual request is allowed or not.
CORS in Modern Applications
In a modern microservices architecture, CORS plays a more complex role than in traditional monolithic applications. When a client application makes a request, it typically first encounters an API Gateway – a central entry point that routes requests to various microservices. The API Gateway usually handles CORS configuration for all underlying services, acting as a unified CORS enforcement point.
This means instead of configuring CORS separately for each microservice (which could lead to inconsistencies and security gaps), the gateway manages all CORS policies. For example, when a React application at app.example.com makes a request to api.example.com, the API Gateway validates the origin and applies the appropriate CORS headers before the request even reaches the target microservice.
This centralized approach simplifies CORS management, ensures consistent policy enforcement, and provides better security control. The gateway can also handle different CORS requirements for different service groups – perhaps allowing broader access to public services while maintaining stricter controls for sensitive operations. Additionally, since internal communication between microservices happens behind the gateway, these services don't need to handle CORS directly, reducing configuration complexity and potential security misconfigurations.

Unleash the power of APIs with Kong Konnect
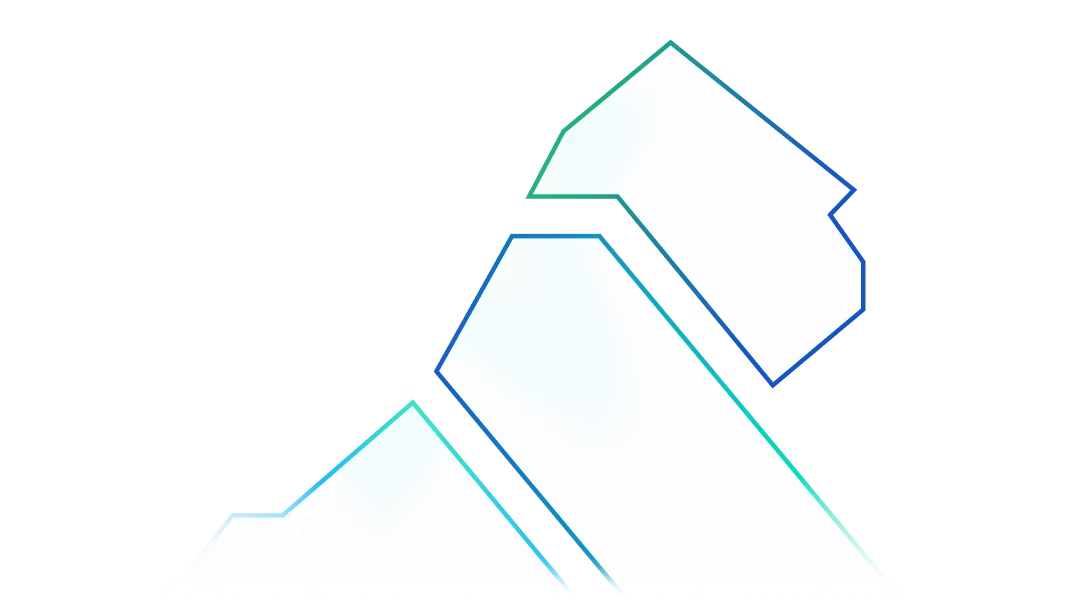
CORS Framework Deep Dive
Now, let's look closely at the technical details of the CORS framework. We will discuss different types of CORS requests and check the headers involved.
Understanding these points will help you set up CORS the right way. It will also help you fix problems during development. When you know these details, you can make sure your web application works well with outside resources, giving users a smooth experience.
Simple vs Preflight Requests
CORS uses two kinds of requests to handle access across different origins: simple requests and preflight requests. Knowing the difference between these two requests is important for safe and effective cross-origin communication.
Simple requests are basic requests that meet certain criteria, making them safer. They usually use common HTTP methods like GET and POST and have limited custom headers, which follow the CORS standards. Because these requests are seen as safe, they don't need a preflight check.
Preflight requests are different. They happen when the browser thinks a request might change data on the server or use complex headers or methods. For example, if the request uses methods like PUT, DELETE, or custom headers that aren't allowed by the CORS settings, it will trigger a preflight request. This serves as a check where the browser asks the server for permission before making the actual request.
Here are the common situations that bring up preflight requests:
- HTTP methods other than GET, HEAD, or POST
- Content-Type headers that are not application/x-www-form-urlencoded, multipart/form-data, or text/plain
- Custom headers that are not in the CORS-safelisted request-headers
Anatomy of CORS Headers
CORS uses certain HTTP headers to manage how different sites talk to each other. These headers help the browser and the server communicate. They set rules about what actions are allowed and how data can be shared.
The key CORS header is the Access-Control-Allow-Origin header. It tells the server which sites can access its resources. The server can set this header to a specific site, like https://www.example.com, or use a wildcard (*). However, using a wildcard is usually not safe because it lets everyone in.
There are other vital CORS headers too, like Access-Control-Allow-Methods, Access-Control-Allow-Headers, and Access-Control-Allow-Credentials. These headers decide which HTTP methods can be used (like GET, POST, and PUT), which custom headers are okay, and if credentials such as cookies or authorization headers can be included with the request.
Common CORS Use Cases and Solutions
CORS setups can be easy, but depending on the level of complexity your needs are, it can become increasingly more complicated. They can do anything from handle simple requests to manage sensitive data with authentication. It is important to identify your specific needs and know the different situations and their solutions, so it becomes easier to use CORS in various development spaces.
In this section, we will look at some typical situations. We will begin with a simple CORS setup. Then, we will move to more complex cases that include authenticated requests. By reviewing these examples, developers can learn how to configure CORS well. This will help them meet their needs and keep cross-origin communication safe.
Basic CORS Setup
Setting up CORS for simple requests is usually easy. Most of the time, you need to configure the server to add the Access-Control-Allow-Origin header to its replies. This header shows which origins can access the server's resources.
For example, to allow requests from a specific site like https://www.example.com, the server should add this header:
Access-Control-Allow-Origin: https://www.example.com
To let requests from any origin, which is not safe, the server can use a wildcard:
Access-Control-Allow-Origin: *
Just adding the header is not enough. The server also needs to let the specific HTTP methods that are used in the request. The web server software, like Apache or Nginx, usually manages this based on its settings.
Handling Authenticated Requests
Handling authenticated requests with CORS can be tricky. The browser must send credentials like cookies or authorization headers to check who you are. By default, browsers do not send these credentials with cross-origin requests unless they are told to do so.
To allow this, the server must add the Access-Control-Allow-Credentials header and set it to true in its response. This tells the browser that the server is ready to accept credentials and will manage them safely.
Access-Control-Allow-Credentials: true
Also, when the server responds to a request with credentials, it needs to clearly list the origin in the Access-Control-Allow-Origin header. Using wildcards is not allowed here. This step ensures that credentials only go to trusted origins.
Even if CORS is set up properly, keeping sensitive data safe depends on strong authentication methods on the server side.
Error Scenarios
Despite careful setup, it's common to face CORS errors during development. These issues often happen because of differences in the CORS headers sent between the browser and the server or incorrect settings on either side. Knowing the usual error types and their reasons can help you find and fix them quickly.
A common error you might see is "CORS origin '…' is not allowed by Access-Control-Allow-Origin." This message means that the server's Access-Control-Allow-Origin header does not include the origin of the website making the request. This may happen because the server's CORS settings are too strict or because the origin request is wrong.
Another frequent error is "Access to XMLHttpRequest … has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource." This error shows that the server is not set up for CORS at all.
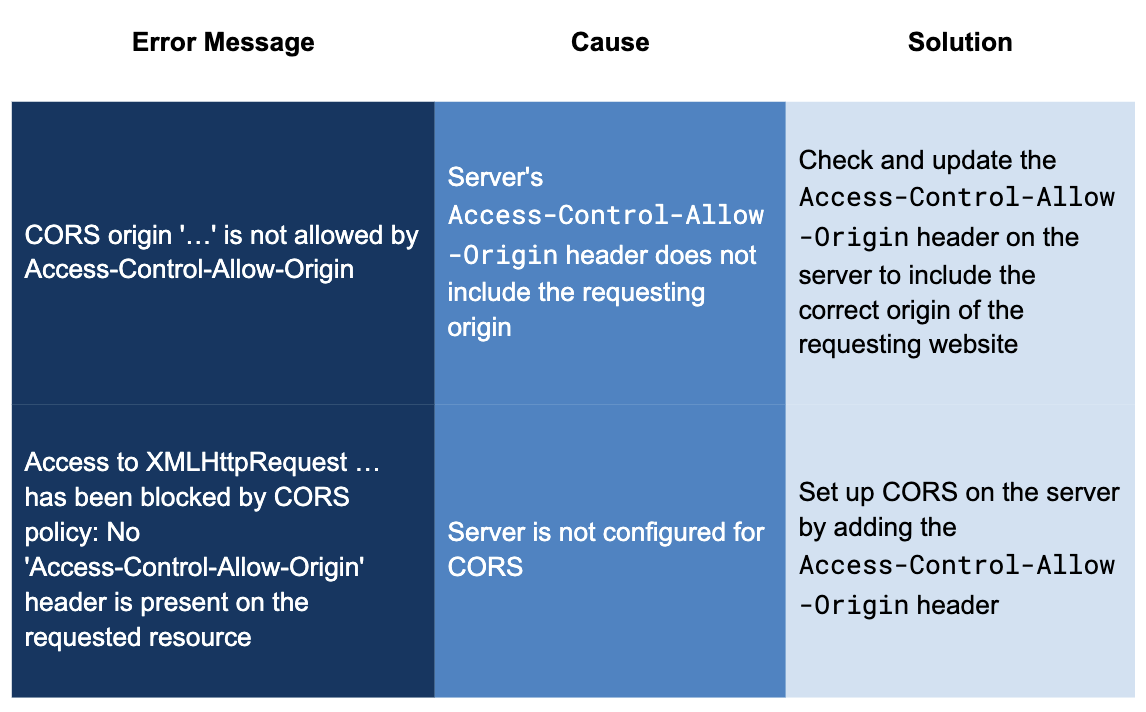
Cross Origin Security Implications
CORS itself is not a threat, but if it is not set up correctly, it can create security problems. Poor CORS settings might weaken the Same-Origin Policy, which could let unauthorized users access your resources.
Keep in mind that CORS is mainly enforced by the browser. It controls how browsers manage requests from different origins. However, it cannot stop bad clients from sidestepping these rules and making direct requests to the server. This means you should not depend only on CORS for security.
CORS Role in Security
CORS is not a security tool by itself, but it is important for improving security in web applications. It controls access from different origins. Its main job is to stop harmful websites from misusing safe websites in cross-origin requests.
One major advantage of CORS is that it helps reduce risks from Cross-Site Scripting (XSS) weaknesses. By allowing only certain origins to access a website’s resources, CORS can stop attackers from using stolen cookies or session tokens from an XSS attack on a weak site. This makes it harder for them to make unauthorized requests to the targeted site.
It's very important to know that CORS needs to be set up correctly to work well. If the settings are too relaxed, like using wildcards in the Access-Control-Allow-Origin header, it can remove the security benefits and make your application exposed to weaknesses. So, following the best security practices and testing your CORS setup properly is very important.
Common Mistakes
One common mistake when setting up CORS is using the Access-Control-Allow-Origin header with a wildcard (*). This is popular but risky, especially for apps with sensitive information. It allows any domain to access your app. This breaks the origin policy and makes your app open to attacks.
Another mistake is not stating the allowed HTTP methods and headers. If you rely on default settings or skip specific methods, you may expose your app to Cross-Site Request Forgery (CSRF) attacks.
Also, not properly checking user input for CORS headers can lead to problems. Bad users might find ways to add harmful origins or change header values. This could help them get around your CORS security. To reduce this risk, you need to validate and clean input carefully.
CORS Implementation Checklist
Successfully using CORS takes thought for both the front end and back end. The details may change depending on your app and tech stack, but there are some general tips that can help make your CORS setup work well and safely.
This checklist shows important things to think about in both front end and back end work. This will help your CORS run correctly and avoid problems with access and security risks.
Frontend Considerations
From the frontend side, making client web applications that connect with external APIs means you need to understand how CORS works on the client side. Most of the CORS setup happens on the server side, but frontend developers must pay attention to some details to avoid problems.
First, make sure that the API requests from your client application include the right headers. Most modern JavaScript frameworks and libraries take care of CORS preflight requests automatically. Still, it's important to check that the correct Origin header is sent with each request. This header tells the server where the request originates.
Second, if your application uses cookies or authentication headers, check that the server allows credentials. You usually do this by setting the withCredentials property to true when making AJAX requests with fetch or XMLHttpRequest. Lastly, test your setup thoroughly with different browsers and devices to make sure everything works the same way on various platforms.
Backend Implementation
Implementing CORS securely and effectively depends on how well you set up your server. You must make your server respond correctly to cross-origin requests. It is important that only allowed sites can access your resources.
First, configure your web server software like Apache or Nginx. You can also use server-side frameworks that already have CORS support. The main part of this setup is to add the right CORS headers, especially the Access-Control-Allow-Origin header. This header tells which sites are allowed.
If your application handles sensitive data and needs user login, you should set the Access-Control-Allow-Credentials header to true. This is necessary if you want to allow credentials such as cookies or authorization headers. But be careful with this setting and check user inputs carefully to avoid security issues. Strong server-side authentication and authorization are key to keeping your data safe. Remember, CORS is meant to support already existing security measures, not replace them.
Let’s Recap
CORS represents a critical balance between security and functionality in modern web development. While it may initially seem like a hurdle, especially during development, CORS serves as an essential security mechanism that protects users and applications from potentially malicious cross-origin requests. By following the best practices outlined in this guide, developers can implement CORS configurations that maintain security without sacrificing functionality. Remember that CORS is just one piece of a comprehensive security strategy – it works best when combined with other security measures like proper authentication, input validation, and secure communication protocols. As web applications continue to become more distributed and interconnected, mastering CORS will remain an essential skill for web developers who want to build secure, efficient, and user-friendly applications.
Frequently Asked Questions
What exactly is CORS?
CORS, which stands for Cross-Origin Resource Sharing, is a way for a web browser to ask for resources from a server that is on a different domain. This means that the server is not the same as the one where the browser loaded the page. It uses special HTTP headers to show what is allowed between the browser and the server.
What's the difference between CORS and API keys?
CORS and API keys have different jobs. CORS, which stands for Cross-Origin Resource Sharing, is a system in the browser. It manages who can access resources from different places. On the other hand, API keys help with authentication. They help identify the application making the request. API keys are not linked to any one specific origin.
Why do I need CORS if I have authentication?
Authentication checks who you are. CORS, which stands for Cross-Origin Resource Sharing, manages access from other websites. Even if you have authentication in place, you still need CORS. It allows approved sites to use resources on your server.
Do I need CORS for my mobile app?
CORS is a security feature used by browsers. Mobile apps that make HTTP requests directly do not follow the same rules as web browsers. Therefore, CORS does not apply to them.